[unity#1] 녹칸다와 함께 내맘대로 유니티(unity) 시리즈를 시작해보기! (녹칸다 디지털트윈/digitaltwin/튜토리얼)
프로그래밍/유니티(unity) 2025. 2. 24. 23:18반응형
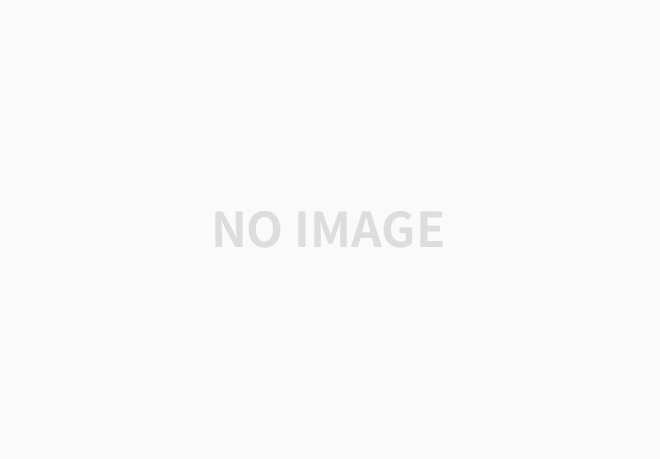
https://youtube.com/live/0U5FTClTmec
[unity#1] 녹칸다와 함께 내맘대로 유니티(unity) 시리즈를 시작해보기! (녹칸다 디지털트윈/digitaltwin/튜토리얼)
녹칸다의 내맘대로 유니티(unity) 시리즈이다!
이번편의 내용은 아래 슬라이드로 공유된다!
https://docs.google.com/presentation/d/1yiia2U7D-iLx6HU1SVTbA4632Rpbq3rOaz8UXqatIcg/edit?usp=sharing
이번편은 녹칸다가 어떻게 유니티 시리즈를 이끌어 나갈지 목표를 설명한다!
그리고 유니티 개발환경을 설정하고 가장 간단히 해볼 수 있는 기본 예시를 제시한다!
(hello world 예제)
helloworld.unitypackage
0.03MB
hello_script.cs
hello_script.cs
0.00MB
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class hello_script : MonoBehaviour
{
//여기에 접근제한자가 public하게 선언된 변수는 유니티 에디터에 노출된다!
public int value = 5;
public Color color1 = Color.yellow;
public Color color2 = Color.red;
// Start is called before the first frame update
void Start()
{
//처음 1회 실행되는 부분
}
// Update is called once per frame
void Update()
{
//매 프레임 반복 실행되는부분
if (Input.GetKeyDown(KeyCode.UpArrow))
{
//이 스크립트가 구에 부여갸 되었는데 구가 가지고 있는 리지드바디를 호출한다!
GetComponent<Rigidbody>().AddForce(1, value, 0, ForceMode.Impulse);
//사용자가 방향키 위쪽을 누르면 눌러졌다고 콘솔창에 출력한다!
print("사용자가 방향키 위쪽을 누름!");
}
else if (Input.GetKeyDown(KeyCode.DownArrow))
{
GetComponent<Rigidbody>().AddForce(-1, value, 0, ForceMode.Impulse);
print("사용자가 방향키 아래쪽을 누름!");
}
else if(Input.GetKeyDown(KeyCode.RightArrow))
{
//구의 포장지인 머테리얼의 컬러를 노란색으로 바꾸겠다!
GetComponent<Renderer>().material.color = color1;
print("사용자가 방향키 오른쪽을 누름!");
}
else if (Input.GetKeyDown(KeyCode.LeftArrow))
{
//구의 포장지인 머테리얼의 컬러를 빨간색으로 바꾸겠다!
GetComponent<Renderer>().material.color = color2;
print("사용자가 방향키 왼쪽을 누름!");
}
else if(Input.GetKeyDown(KeyCode.Q))
{
//프로그램종료
Application.Quit();
}
}
//이벤트
private void OnCollisionEnter(Collision collision)
{
//object(this)에 뭔가 충돌이 발생했다!
//색상을 바꾸겠다!
//여기서 큐브와 충돌되었다라고 하려면 큐브의 object를 찾아야한다!
//큐브에다가 tag값을 입력해주고 tag값으로 감별한다!
if (collision.gameObject.tag == "mybox")
{
GetComponent<Renderer>().material.color = color1;
print("박스와 구가 충돌됨!");
}
}
private void OnCollisionExit(Collision collision)
{
//object(this)에 뭔가 충돌이 되었다가 접촉이 해제되었다!
//색상을 바꾸겠다!
if (collision.gameObject.tag == "mybox")
{
GetComponent<Renderer>().material.color = color2;
print("박스와 구가 충돌이 되었다가 떨어짐!");
}
}
}
hello_script2.cs
hello_script2.cs
0.00MB
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class hello_script2 : MonoBehaviour
{
//이 스크립틑 박스 object에 줄 예정이다!
//이 컴포넌트의 this는 박스 object가 된다!
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//사용자가 숫자키 1을 입력하면 박스가 0,0,0으로 이동한다
if(Input.GetKeyDown(KeyCode.Alpha1)) {
transform.position = new Vector3(0, 0, 0);
}
//사용자가 숫자키 2를 입력하면 박스가 3,0,0으로 이동한다
if (Input.GetKeyDown(KeyCode.Alpha2))
{
transform.position = new Vector3(3, 0, 0);
}
//사용자가 숫자키 3를 입력하면 박스가 0,3,0으로 이동한다
if (Input.GetKeyDown(KeyCode.Alpha3))
{
transform.position = new Vector3(0, 3, 0);
}
}
}
hello_script3.cs
hello_script3.cs
0.00MB
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using static UnityEngine.GraphicsBuffer;
public class hello_script3 : MonoBehaviour
{
Vector3 Target = new Vector3(0,0,0); //목표지점
public float Speed = 1f; //이동 속도
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
//키보드4를 누르면 3,0,0으로 이동한다!
if(Input.GetKeyDown(KeyCode.Alpha4))
{
Target = new Vector3(3, 0, 0);
}
//키보드 5를 누르면 0,3,0으로 이동한다!
if (Input.GetKeyDown(KeyCode.Alpha5))
{
Target = new Vector3(0, 3, 0);
}
transform.position = Vector3.MoveTowards(transform.position, Target, Speed * Time.deltaTime);
}
}
hello_script4.cs
hello_script4.cs
0.00MB
using System.Collections;
using System.Collections.Generic;
using Unity.VisualScripting;
using UnityEngine;
public class hello_script4 : MonoBehaviour
{
//에디터에 노출되고 에디터에서 해당되는 object를 선택해주면된다!
public GameObject mysphear; //null
public GameObject mybox; //null
public GameObject mycylinder; //null
Material mysphear_material;
Material mybox_material;
Material mycylinder_material;
// Start is called before the first frame update
void Start()
{
mysphear_material = mysphear.GetComponent<Renderer>().material;
mybox_material = mybox.GetComponent<Renderer>().material;
mycylinder_material = mycylinder.GetComponent<Renderer>().material;
}
// Update is called once per frame
void Update()
{
//방법1
//이 스크립트에서 this는 바로 빈오브젝트이다!
//this.GetComponent<Rigidbody>()
if (Input.GetKeyDown(KeyCode.Alpha6))
{
//3개의 object가 모두 녹색이된다
mysphear_material.color = Color.green;
mybox_material.color = Color.green;
mycylinder_material.color = Color.green;
}
if (Input.GetKeyDown(KeyCode.Alpha7))
{
//3개의 object가 모두 빨간색이된다!
mysphear_material.color = Color.red;
mybox_material.color = Color.red;
mycylinder_material.color = Color.red;
}
}
}
반응형